This post covers the following points:
- How to open the project properties dialog box for NetBeans IDE projects?
- How to use command line arguments while running programs through NetBeans IDE?
NetBeans IDE provides a way to invoke our Java program having a main method with command line arguments. Let us walk through this process. We will create a new Java Application project and will add some code to the Main method which will print the provided command line arguments on the console.
Test Code we have written in the Main method:
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package com.tusharvjoshi.argsdemo;
/**
*
* @author Tushar Joshi
*/
public class Main {
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
if (args.length == 0) {
System.out.println("No Command Line arguments");
} else {
System.out.println("You provided " + args.length
+ " arguments");
for (int i = 0; i < args.length; i++) {
System.out.println("args[" + i + "]: "
+ args[i]);
}
}
}
}
Opening the project properties dialog boxWe can either right click on the project name in the Projects panel and choose the properties option, or we can choose the Project Properties option from the File menu.
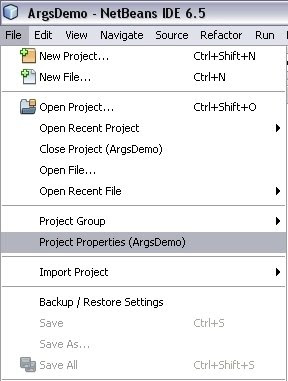
Saving the command line arguments
In the project Properties dialog box we will have to choose the Run node from the left Categories tree. As soon as we select the Run node we can see the options for the run configuration.
In the Arguments text box we have to type our command line arguments. We can see we have typed "one two three" as the options in the dialog box for testing.
Now when we Run the project the project will run as if these command line arguments are being typed in front of the java command. We can see the output window to check how the arguments are printed by our test code.
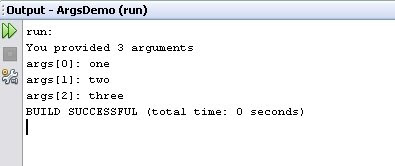
with regards
Tushar Joshi, Nagpur
UPDATE: 21 Mar 2014:
There is a plugin available for NetBeans IDE 8.0 which allows interactive command line arguments facility to the IDE, more details are in this post
UPDATE: 21 Mar 2014:
There is a plugin available for NetBeans IDE 8.0 which allows interactive command line arguments facility to the IDE, more details are in this post
I've typed this in exactly but debugging the app does not pass any of the command-line options into args[]. What is the problem?
ReplyDeleteYou may have had the same problem that I had...
ReplyDeleteThe path to your run-time command line args is stored in the netbeans private.properties file
as "application.args=".
You may have overridden your private.properties file with an entry in your build.xml of
target name="-init-private" depends="-pre-init"
If you have, then args[] won't get picked up at runtime unless you create an overriding entry in your project.properties file to replace the one that should be picked up from your private.properties.
Hope this might help
where should we find and change this error?
DeleteWhere can i make the above changes?
DeleteThank alot it's very useful
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteI have tried everything above and nothing works.
ReplyDeleteThe code above returned "No Command Line Arguments". Anyone have any ideas?
do the configuration and try i had same problem but after doing the configuration it works
Deletehey its working...
ReplyDeletethank u very much
thanks, it's working
ReplyDeleteEven if the topic of this post is very trivial, I can see that some people got benefited by reading it.
ReplyDeleteThanks all for the good words.
with regards
Tushar Joshi
hi. I am not able to pass the command line arguments to my app? what could be the problem????
ReplyDeleteHi, i essentially want to do the same thing, but instead of just words like one, two, and three. I want to pass a text file, thats inside the same package. Can anyone help?
ReplyDeleteThanks
You can very well pass the path and name of the file as a command line parameter and use that file in your program.
ReplyDeleteIf you keep your file in the same package then the getResource method in the class loader can be used.
with regards
Tushar
Thanks a lots its working.....
ReplyDeleteif you do not have args picked up properly even though you formate it from project's run arguments, you can try to change "run" target in build-impl.xml to say
ReplyDeletehow can i pass command line arguments when i have too many main in my package?
ReplyDelete@Shresth,
ReplyDeleteIn the same doalog box where you mention command line arguments you will see Main Class as the input box, you will have to type the main class which you want to run.
I believe you already know that we can execute only ONE main class at a time through one Java command.
with regards
Tushar Joshi, Nagpur
thank you so much for the help!
ReplyDeleteits really useful..thanks a lot
ReplyDeletethanx tushar
ReplyDeletethanks
ReplyDelete@malor: I am facing the same issue mentioned by you.
ReplyDeleteI am able to add the arguments through the interface project properties option but it doesn't get picked up. When I check the argument in netbeans private.properties file it comes as
"application.args= -3.0 2.0" but nothing happens. It still gives my java.lang.ArrayIndexOutOfBoundsException
I didn't understand how to override the entry in build.xml to get everything back to normal.
please help me out if any one has any idea.
Thanks
ThankYOU! ThankYOU! ThankYOU! ThankYOU! ThankYOU! ThankYOU! GOD BLESS YOU !
ReplyDeletethanks..
ReplyDeleteNot working... N i also checked project.properties file.. I havent overridden application.args
ReplyDeletePlz help..
@Gunit,
ReplyDeleteIf you provide more details like:
1) Which version of NetBeans IDE are you using?
2) What is not working? Is there any error message you are getting? Can you share that message here
3) What is your application scenerio? Are you following the exact code given in this article or something else?
I may help you if these details are known.
with regards
Tushar Joshi, Nagpur
Thanks... The post is quiet explanatory and easy.
ReplyDeleteI'm trying to send file path Through main method
ReplyDeleteand I did exactly like what you did
but it still not working
it gives me this error message
Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: 0
at Security.main(Security.java:277)
Java Result: 1
what can I do to solve this problem ?
Thank You
i cant find label "arguments" and its "textbox" where i can put the values.. how can this happen?
ReplyDeleteOk. The post is completely correct. :)
ReplyDeletevery useful. Thanx...
ReplyDeleteWhen I input text files into the arguments field, I get FileNotFoundException yet I have the text files saved into the same package! I'm stumped, can someone help me!
ReplyDeleteHi Tushar,
ReplyDeleteThanks for the upload showing how to pass the command line argument in Netbeans.
I did the same thing but still no luck. however i am able to run my program on command prompt but not on NetBeans(Product Version: NetBeans IDE 7.1.2 (Build 201204101705). Any Suggestions please?
hey its working thank you tushar
ReplyDeletedo as stated .
ReplyDeleteThen run the main project(not class) OR press f6 .
Thanks Tushar. Well explained, very useful for newbies like us.
ReplyDeleteThanks a lot buddy, cheers :)
ReplyDeleteThanks a lot, worked for me when I click on the visual button to run the main method, but When I use Shift-F6 it doesnt, hope it will be hepful for some folks :)
ReplyDeleteSame with me... But why?
Deletethanks a lot, it's working thank you very much
ReplyDeletethank you so much:)
ReplyDeleteThank you very much!!! I was having so many problems with this!!
ReplyDeleteThank you so much! Best wishes for you!
ReplyDeleteThe Answer is easy in fact :
ReplyDelete1-right click in the name of project like "myFirstProject".
2-choose ------> properties.
3-choose ------>Run.
4-in the argument text field put your args like----------
one two three
here we put 3 argument
5- every body do the same above but the magic touch is ------> right click in the "name of project" which is in our case "myFirstProject"
and choose --------> Run
like that the args will work,
but if you gone to main class and choose right click then choose ---> Run the args will not work.
If you want to run any file with arguments, you may like to take a look at the plugin RunWithArgs mentioned in this post http://netbeanside61.blogspot.in/2014/03/command-line-arguments-for-java.html
Deletethank you Tushear , but the plug in in the above url just work with JDK 8 only
DeleteNot JDK8.0 this plugin works with NetBeans IDE 8.0 which is the latest version and everyone who can must shift to the latest version of NetBeans IDE. It also runs on Java 7.0
Deletethanks
DeleteTanks a lot, it works.
DeleteMounir NAJAHI
Thank you so much
ReplyDeleteThanks tushar...thanks alot...
ReplyDelete